🔗 문제 링크
https://school.programmers.co.kr/learn/courses/30/lessons/17687
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
👩💻 코드
#include <string>
#include <vector>
#include <algorithm>
#include <iostream>
using namespace std;
string decimalToBase(int base, int number){
if(number == 0){
return "0";
}
string result = "";
string digits = "0123456789ABCDEF";
while (number > 0) {
result += digits[number % base];
number /= base;
}
reverse(result.begin(), result.end());
return result;
}
string solution(int n, int t, int m, int p) {
string answer = "";
string numbers = "";
int i = 0;
while(numbers.size() < t * m){
numbers += decimalToBase(n, i);
i++;
}
for (int j = 0; j < t; j++) {
answer += numbers[(p - 1) + j * m];
}
return answer;
}
📝 풀이
우선 10진수를 원하는 진법에 맞게 변환해주는 함수(decimalToBase)를 구현했습니다.
0인 경우는 예외처리를 하고, 16진법까지 나타내야 함으로 digits을 새로 정의하였습니다.
number가 0보다 클 때까지 나눗셈을 반복하며 각 자릿수의 숫자를 구합니다.
변환 결과는 역순이기 때문에 reverse함수를 사용하여 문자열을 뒤집어줍니다.
튜브가 말할 숫자의 개수를 충분히 구할 수 있도록 변환된 숫자를 numbers에 추가합니다.
변환된 숫자의 문자열 numbers에 p번째 사람이 말할 숫자만 answer에 추가합니다.
p번째는 index보다 1이 크기때문에 p - 1 를 해야합니다.
최종적으로 answer에는 튜브가 말해야 하는 숫자가 담겨져 있으니 return 합니다.
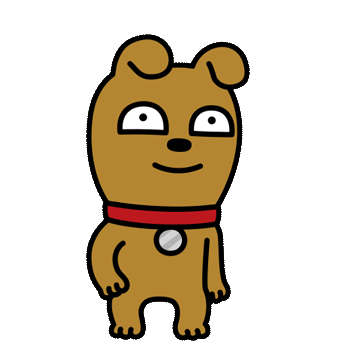
'코딩테스트 > 프로그래머스' 카테고리의 다른 글
[프로그래머스] 게임 맵 최단거리 (0) | 2024.08.14 |
---|---|
[프로그래머스] k진수에서 소수 개수 구하기 (1) | 2024.08.12 |
[프로그래머스] 타겟 넘버 (0) | 2024.08.12 |
[프로그래머스] [1차] 뉴스 클러스터링 (0) | 2024.08.08 |
[프로그래머스] 피로도 (0) | 2024.08.03 |